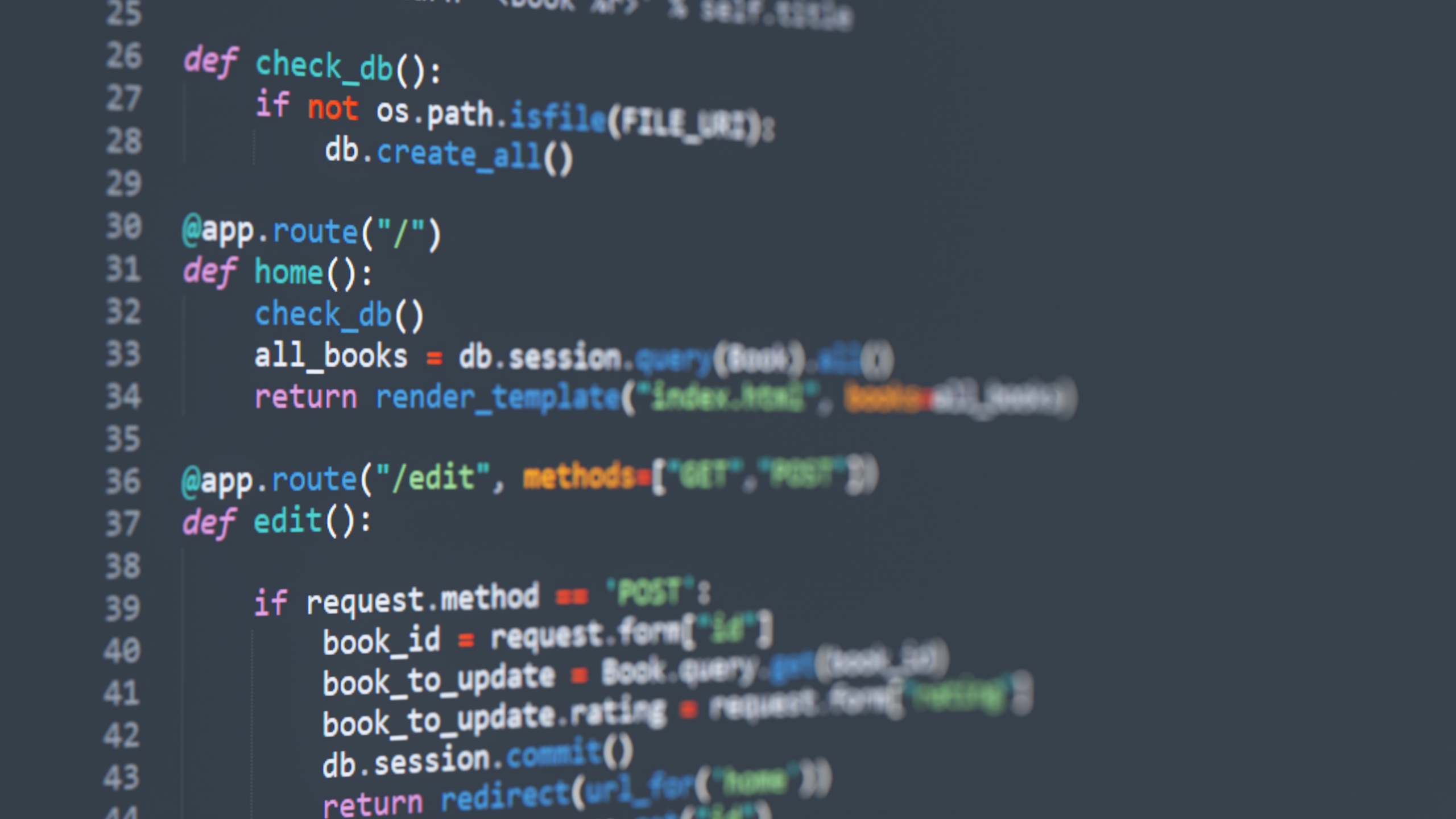
Writing code for online games, particularly for mobile or web-based slot games like this one, involves a mix of advanced graphics rendering, real-time networking, security implementations, and intricate game logic. Developers need to balance between performance optimization, cross-platform compatibility, and the unique requirements of the online gambling industry, such as randomization and fairness.
In this article, we’ll dive into more technical details about the specific challenges and include code examples to illustrate key areas of game development for both multiplayer online games and casino slot games.
1. Performance Optimization: Rendering and FPS Management
For both online and slot games, frame rate stability and resource management are critical to ensure that games perform smoothly across devices.
Game Loop Example
The core of any real-time game is the game loop, which handles rendering, physics, and user input. In JavaScript (often used for HTML5 games like slot machines), a basic game loop looks like this:
let lastTime = 0;
function gameLoop(time) {
const deltaTime = time - lastTime;
lastTime = time;
update(deltaTime); // Updates game logic, like slot spins or player movement
render(); // Redraws the canvas
requestAnimationFrame(gameLoop); // Recursively calls the game loop
}
requestAnimationFrame(gameLoop);
In high-performance games, optimization techniques like object pooling (reusing memory to avoid frequent allocations) and culling (only rendering what is visible) are essential. This helps in avoiding performance bottlenecks, especially when rendering complex 3D environments.
Example: GPU Acceleration
To achieve high frame rates, many games leverage WebGL or Metal (iOS) for hardware-accelerated rendering. In a game like a slot machine, developers can use WebGL shaders to achieve smooth animations for spinning reels.
// GLSL code for fragment shader (example for a reel's background in slot games)
precision mediump float;
uniform vec2 u_resolution;
void main() {
vec2 uv = gl_FragCoord.xy / u_resolution.xy;
gl_FragColor = vec4(uv.x, uv.y, 0.5 + 0.5 * sin(uv.x * 10.0), 1.0);
}
This shader code uses the x-coordinates of the screen to create a dynamic background effect on a slot machine reel.
2. Random Number Generation and Fairness
Fairness in online slot games revolves around Random Number Generators (RNGs). RNGs ensure that every spin outcome is independent and random, a core requirement for casino games.
Basic RNG in JavaScript for a Slot Machine
An RNG implementation can be as simple as JavaScript’s Math.random()
function:
function getRandomSlotSymbol() {
const symbols = ["Cherry", "Bell", "Lemon", "Seven"];
const randomIndex = Math.floor(Math.random() * symbols.length);
return symbols[randomIndex];
}
// Example: Spin 3 reels
console.log(getRandomSlotSymbol(), getRandomSlotSymbol(), getRandomSlotSymbol());
For real-world implementations, the RNG needs to be cryptographically secure. Libraries like crypto in Node.js can be used for a more secure RNG.
const crypto = require('crypto');
function getSecureRandomSymbol() {
const symbols = ["Cherry", "Bell", "Lemon", "Seven"];
const randomIndex = crypto.randomInt(0, symbols.length);
return symbols[randomIndex];
}
3. Multiplayer Synchronization and Networking
In multiplayer online games, synchronizing actions between players in real-time is crucial. This typically involves the client-server model, where the server holds the game’s state and clients send input to be processed by the server.
Example: Client-Server Sync in Node.js (WebSockets)
Using WebSockets, we can send and receive real-time messages between the client and server to synchronize player movements in a game.
// Server-side code (Node.js with WebSocket)
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
let players = {};
wss.on('connection', (ws) => {
ws.on('message', (message) => {
const data = JSON.parse(message);
players[data.id] = data.position;
broadcastToClients(data);
});
ws.on('close', () => {
// Handle player disconnect
});
});
function broadcastToClients(data) {
wss.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(JSON.stringify(data));
}
});
}
On the client-side, the game sends its player’s position to the server, which then broadcasts it to other connected clients.
// Client-side (JavaScript for browser)
const ws = new WebSocket('ws://localhost:8080');
ws.onmessage = function(event) {
const data = JSON.parse(event.data);
updatePlayerPosition(data.id, data.position);
};
// Sending player position to server
function sendPlayerUpdate(id, position) {
const data = { id, position };
ws.send(JSON.stringify(data));
}
This pattern is essential in multiplayer environments where players’ actions (like movement or combat) need to be reflected instantly on all clients.
4. Cross-Platform Development
With the diversity of platforms, developers aim to write once and deploy everywhere. Game engines like Unity and frameworks like Phaser.js (for 2D games) are used for cross-platform compatibility.
In slot games, HTML5 is often the preferred technology due to its wide support across web and mobile platforms. Here’s a simplified Phaser.js example of how a slot reel might be implemented:
const config = {
type: Phaser.AUTO,
width: 800,
height: 600,
scene: {
preload: preload,
create: create,
update: update
}
};
const game = new Phaser.Game(config);
function preload() {
this.load.image('cherry', 'assets/cherry.png');
this.load.image('bell', 'assets/bell.png');
}
function create() {
const symbols = ['cherry', 'bell'];
const reel = this.add.sprite(400, 300, symbols[Phaser.Math.Between(0, symbols.length - 1)]);
// Simulate spinning reel
this.time.addEvent({
delay: 1000,
callback: () => reel.setTexture(symbols[Phaser.Math.Between(0, symbols.length - 1)]),
loop: true
});
}
function update() {
// Game update logic here
}
This code sets up a basic Phaser.js game where a slot reel randomly switches between two symbols every second.
5. Monetization and In-App Purchases
Monetization is central to online games, particularly mobile games and slots. In-app purchases and currency systems are coded to allow players to buy additional spins, power-ups, or cosmetic items.
For mobile apps, Google Play’s Billing Library or Apple’s StoreKit are used to handle transactions securely. An example flow might look like this:
// Example purchase function for Google Play In-App Billing
function initiatePurchase(productId) {
// Start a purchase flow using Google Play Billing API
billingClient.launchBillingFlow(activity, BillingFlowParams.newBuilder()
.setSkuDetails(skuDetailsMap.get(productId))
.build());
}
// Callback function after purchase
billingClient.setListener(new PurchasesUpdatedListener() {
@Override
public void onPurchasesUpdated(int responseCode, List<Purchase> purchases) {
if (responseCode == BillingClient.BillingResponseCode.OK && purchases != null) {
// Grant the player the in-game currency or item
grantInGameCurrency(purchases);
}
}
});